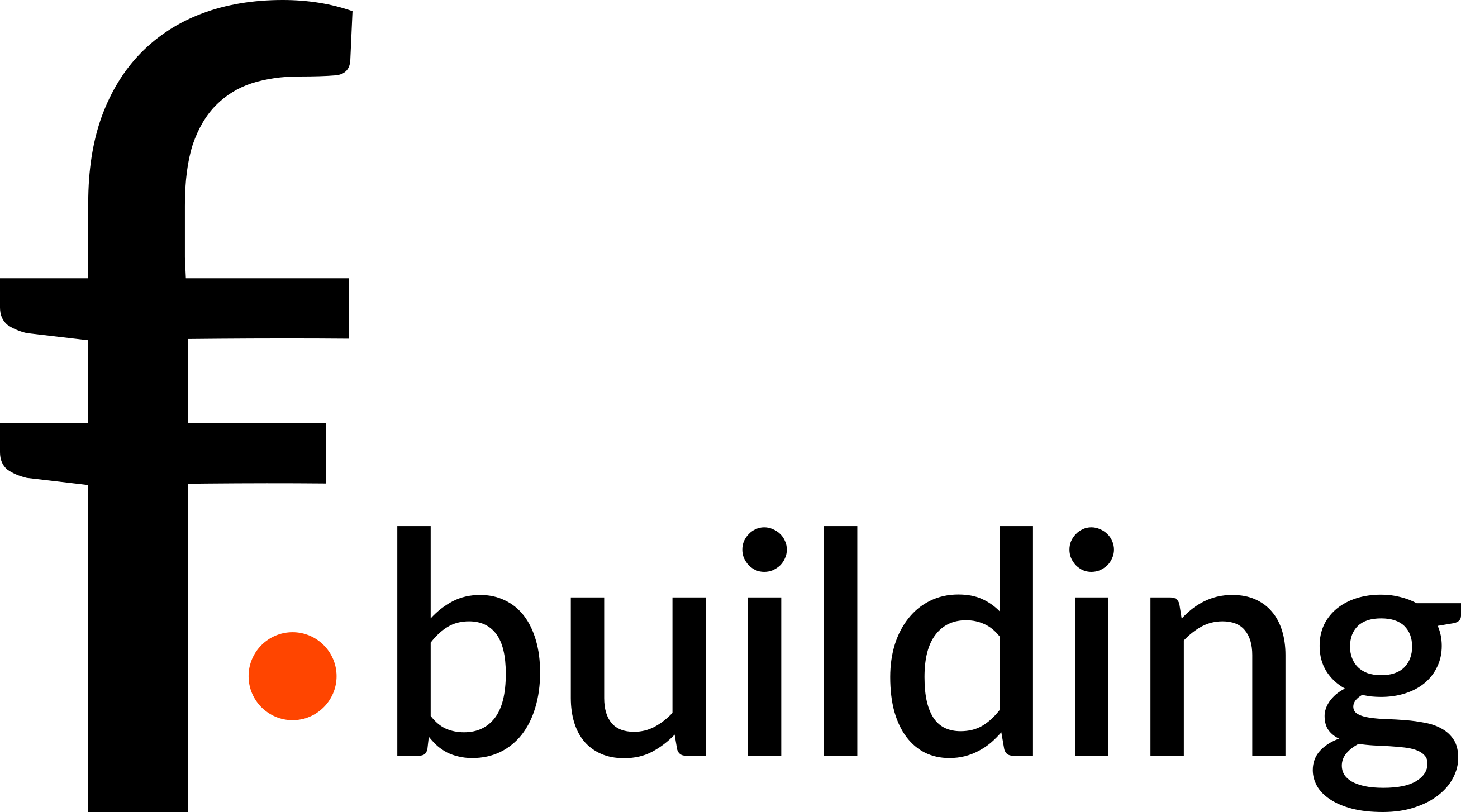
Simulating the hydraulic behavior of the interior of a building
David Nortes Martínez
hydraulic_model.Rmd
Basic workflow
Set up
This vignette is going to help you simulate the hydraulic behavior of
the interior of a building using floodam.building
. To do so
you need 3 key inputs:
- The surface and floor level of each room
- The connections between two rooms as well as the connections with the exterior of the buildings. Hereafter we will refer to these connections as exchanges
- The evolution of the floodwater depth in front of each opening connecting the exterior with the interior of the building as well as the initial floodwater depth in the interior of the building (usually 0)
The library floodam.building
provides methods to
calculate each of these elements. Let us see how.
To determine the two first key inputs, the function
analyse_model()
provides you with the stage
hydraulic. We are using one of the models shipped with with
floodam.building
to make this example:
- The models are available in your library’s installation folder. To make them available, just ask R to locate them:
# set up model to use example shipped with floodam
model_path = list(
data = system.file("extdata", package = "floodam.building"),
output = tempdir()
)
-
We are going to use the model called adu_t. This model proposes a 4-room house where three of them are organized around a central living room. As stages of analysis we are going to specify
c("load", "extract", "hydraulic")
. If you check the objectmodel
, you can see that inside the slot called hydraulic there are four different slots containing three data.frames:- exchanges open
- exchanges_close
- exchanges_combined
- rooms
The data.frame rooms corresponds to the first key inputs, while the data.frames exchanges open, exchanges_close and exchanges_combined are variants of the second key input: all openings open, all openings closed but not waterproof, and all exterior openings closed and all interior openings open.
library(floodam.building)
model = analyse_model(
model = "adu_t",
type = "adu",
stage = c("load", "extract", "hydraulic"),
path = model_path
)
-
To simulate the third key input,
floodam.building
provides the functiongenerate_limnigraph()
. This function generates a limnigraph, i.e. the evolution of floodwater depth against time. You need to manually provide different parameters to this function:- time: vector of time steps in seconds. Usually it contains three elements: the initial time step, the time step where water outside the building is at its peak, and the final time step. In the example provided this time steps are 0, 5400 and 10800 seconds.
- depth: vector of floodwater depth in meters. This vector contains as many elements as the vector of time steps. It indicated the water depth in each time step.
- exposed_openings: openings communicating the exterior of the building with the interior and that are exposed to the flood event. In the example provided, three out of the four openings that communicate the exterior with the interior of the building are assumed to be exposed to this particular flood event.
The function returns a data.frame with time steps as rows and openings as columns.
flood = generate_limnigraph(
model = model,
time = c(0, 5400, 10800),
depth = c(0,3,0),
exposed_openings = c("door1", "window1", "window2")
)
Simulate hydraulics
Once the three key parameters are determined, the simulation of the
hydraulic behavior of the building for the flood event designed can be
simulate. To do so, floodam.building
provides the function
analyse_hydraulics()
. This function takes three mandatory
parameters:
-
model: the output of the function
analyse_model()
(see above) -
limnigraph: the output of the function
generate_limnigraph()
(see above) - opening_scenario: One of the following options: open, closed, combined or user_def. If you choose this last option, the additional parameter exchange should be provided.
Additionally, the function can be provided with the parameter sim_id, in order to properly identify the simulation when working within an experimental design.
output_hydrau = analyse_hydraulics(
model = model,
limnigraph = flood,
opening_scenario = "close",
sim_id = "integrated_model"
)
The function returns a list with four slots for four data.frames:
- evolution of floodwater depth in each room
- evolution of the discharge volume through each opening
- evolution of the discharge section through each opening
- evolution of the discharge velocity (calculated with the volume and section data) through each opening