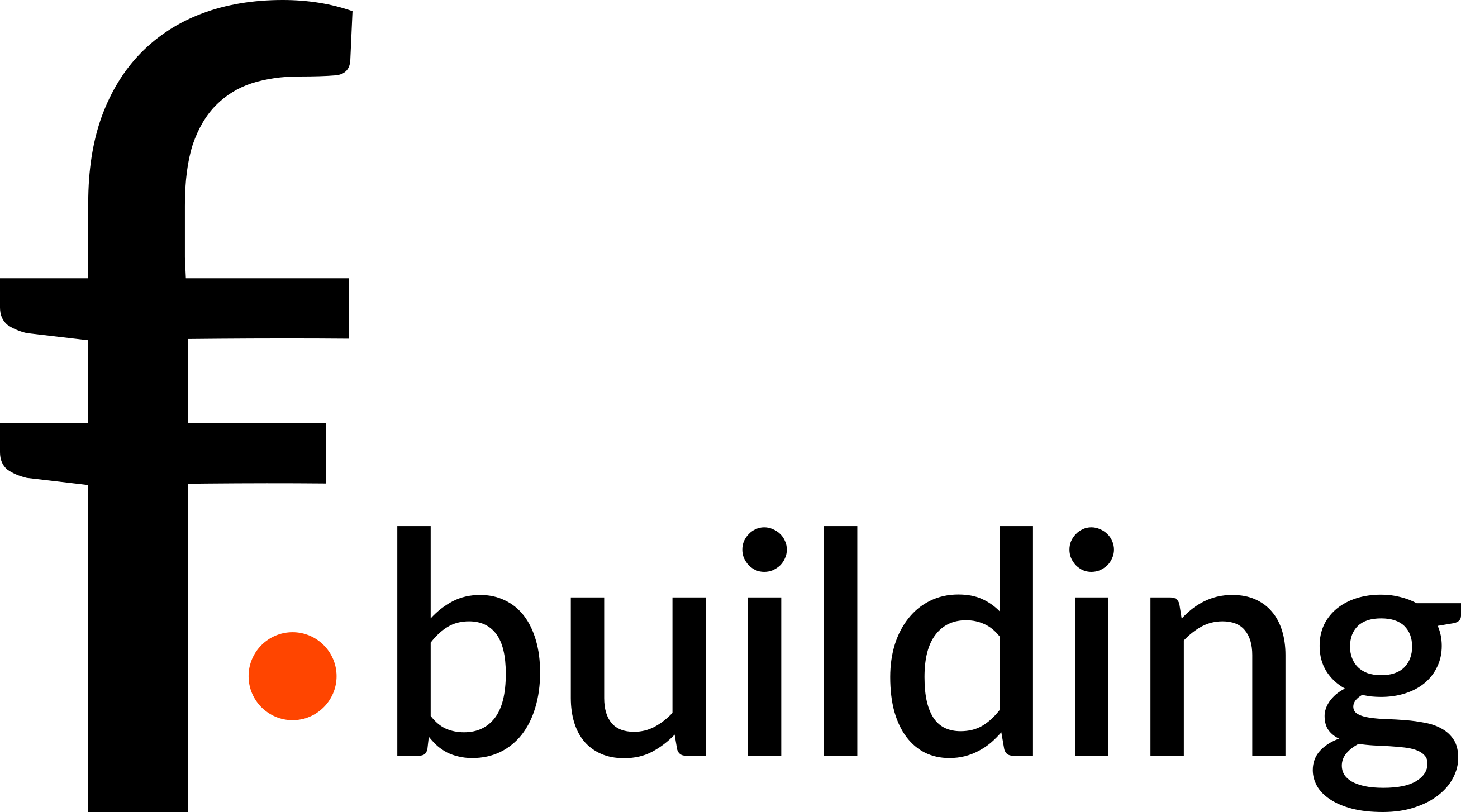
Perfom hydraulic analysis for a model
analyse_hydraulic.Rd
analyse_hydraulic()
perfoms hydraulic analysis for a model.
Arguments
- model
either a character, name given to the model or an object of class model
- limnigraph
numeric, matrix of floodwater depth heights by instant t for each exterior opening exposed to flooding
- flood_duration
numeric, duration of the flood event in seconds, optional; if not provided, calculated using limnigraph
- opening_scenario
character, one of the following options: "open", "closed", "combined". See details.
- dt_max
numeric, optional; maxmimum timestep for simulation
- Cd
numeric, optional; discharge coefficient for each opening, default to getOption("floodam_building_hydraulic_discharge_coefficient")
- sim_id
character, optional; id of simulation if function called from a loop
- stage
character, what are the stages that should be done, default to nothing
- what
character, outputs to be saved
- verbose
boolean, will floodam tells what it is doing, default to getOption("floodam_building_verbose")
Value
list of matrix:
h
: water depths in each rooms for each time stepeQ
: water exchanges through openings for each time stepv
: velocity of exchanges through openings for each time stepeS
: wet surfaceof exchanges through openings for each time step
Details
opening_scenario
controls the state of openings:
"open": scenario where all openings are open
"closed": scenario where all openings are closed
"combined": scenario where all exterior openings are closed and all interior openings are open
If flood_duration
is missing, it is computed from limnigraph
(max time +
half an hour).
Examples
# declaring input and output paths
model_path = list(
data = system.file("extdata", package = "floodam.building"),
output = tempdir()
)
# analyzing model 'adu_t' of type 'adu' using the sequential steps *load*
# (load model data), *extract* (extract model data) and *hydraulic*
model = analyse_model(
model = "adu_t",
type = "adu",
stage = c("load", "extract", "hydraulic"),
path = model_path
)
#> Loading model 'adu_t'...
#> - Structure of building.xml of 'adu_t' has been successfully checked
#> ... successful
#> Extracting building information for 'adu_t'...
#> - extracted:
#> - parameter
#> - storey
#> - room
#> - wall
#> - opening
#> - coating
#> - furniture
#> - missing (not found):
#> ... Informations successfully extracted for 'adu_t'
#> Computing some values for 'adu_t'...
#> ... Informations successfully extracted for 'adu_t'
#> Extracting input data for hydraulic model for 'adu_t'...
#> ... converting hydraulic input data in 'adu_t' to meters
#> ... hydraulic input data in 'adu_t' succesfully converted to meters
#> ... hydraulic input data successfully extracted for 'adu_t'
#> End of analysis for 'adu_t'. Total elapsed time 0.34 secs
#> More information availabe at /tmp/Rtmprv2Zow/model/adu/adu_t/adu_t.log
#' # generate limnigraph
limnigraph = generate_limnigraph(
time = c(0, 5400, 10800),
depth = cbind(external_1 = c(0, 3, 0), external_2 = c(0, 0, 0)),
external = list(
external_1 = c("door1", "window1", "window2"),
external_2 = c("window3")
)
)
#> generating limnigraph ...
#> limnigraph successfully generated
hydraulic = analyse_hydraulic(
model = model,
limnigraph = limnigraph,
sim_id = "test",
stage = "hydraulic"
)
#> Simulating hydraulics for 'adu_t'...
#> ... hydraulics successfully modeled for 'adu_t'
#> End of analysis for 'adu_t'. Total elapsed time 1.42 secs